在 word 文档中,内容控件使得文档的内容可以动态地更新和修改,为用户提供了更灵活的编辑和管理选项。通过内容控件,用户可以轻松地插入、删除或修改特定部分的内容,而无需改变文档的整体结构。本文将介绍如何使用 spire.doc for .net 在 c# 项目中修改 word 文档中的内容控件。
安装 spire.doc for .net
首先,您需要将 spire.doc for .net 包含的 dll 文件作为引用添加到您的 .net项目中。dll 文件可以从此链接下载,也可以通过 安装。
pm> install-package spire.doc
c# 修改正文内容控件
在 spire.doc 中,正文内容控件的对象类型是 structuredocumenttag。您可以通过遍历 section.body 中的子对象集合,查找到类型为 structuredocumenttag 的对象,然后对其进行修改。以下是详细的步骤:
- 创建一个 document 对象。
- 使用 document.loadfromfile() 方法加载一个文档。
- 使用 section.body 获取文档一个节的正文部分。
- 遍历正文部分的子对象集合 body.childobjects,获取到类型为 structuredocumenttag 的子对象。
- 进入 structuredocumenttag.childobjects 子对象集合,根据子对象的类型执行相应的修改操作。
- 使用 document.savetofile() 方法保存到文档。
- c#
using spire.doc;
using spire.doc.documents;
using system.collections.generic;
namespace spirexlsdemo
{
internal class program
{
static void main(string[] args)
{
// 创建一个新的文档对象
document doc = new document();
// 从文件加载文档内容
doc.loadfromfile("示例1.docx");
// 获取文档正文部分
body body = doc.sections[0].body;
// 创建段落列表和表格列表
list paragraphs = new list();
list tables = new list
();
for (int i = 0; i < body.childobjects.count; i )
{
// 获取文档对象
documentobject documentobject = body.childobjects[i];
// 如果是structuredocumenttag对象
if (documentobject.documentobjecttype == documentobjecttype.structuredocumenttag)
{
structuredocumenttag structuredocumenttag = (structuredocumenttag)documentobject;
// 如果标签为"c1"或者别名为"c1"
if (structuredocumenttag.sdtproperties.tag == "c1" || structuredocumenttag.sdtproperties.alias == "c1")
{
for (int j = 0; j < structuredocumenttag.childobjects.count; j )
{
// 如果是段落对象
if (structuredocumenttag.childobjects[j].documentobjecttype == documentobjecttype.paragraph)
{
paragraph paragraph = (paragraph)structuredocumenttag.childobjects[j];
paragraphs.add(paragraph);
}
// 如果是表格对象
if (structuredocumenttag.childobjects[j].documentobjecttype == documentobjecttype.table)
{
table table = (table)structuredocumenttag.childobjects[j];
tables.add(table);
}
}
}
}
}
// 修改第一个段落的文本内容
paragraphs[0].text = "成都冰蓝科技有限公司致力于为开发人员提供 .net 和 java 组件开发产品。";
// 重置第一个表格的单元格为5行4列
tables[0].resetcells(5, 4);
// 将修改后的文档保存到文件
doc.savetofile("修改word文档正文内容控件.docx", fileformat.docx2016);
// 释放文档资源
doc.dispose();
}
}
}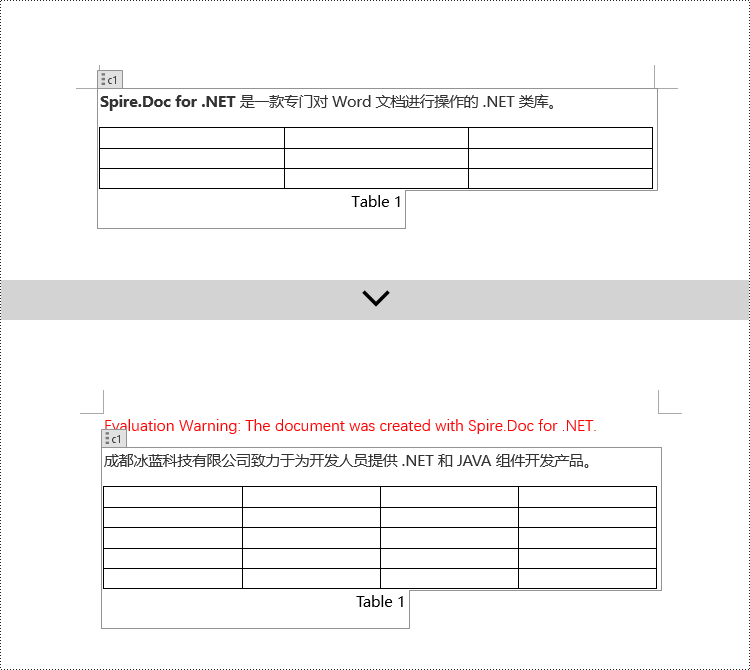
c# 修改段落中的内容控件
在 spire.doc 中,段落中的内容控件对象类型是 structuredocumenttaginline。需要遍历 paragraph.childobjects 的子对象集合,查找到类型为 structuredocumenttaginline 的对象,然后对其进行修改。以下是详细的步骤:
- 创建一个 document 对象。
- 使用 document.loadfromfile() 方法加载一个文档。
- 使用 section.body 获取文档一个节的正文部分。
- 使用 body.paragraphs[0] 获取正文部分的第一个段落。
- 遍历段落的子对象集合 paragraph.childobjects,获取到类型为 structuredocumenttaginline 的子对象。
- 进入 structuredocumenttaginline.childobjects 子对象集合,根据子对象的类型执行相应的修改操作。
- 使用 document.savetofile() 方法保存到文档。
- c#
using spire.doc;
using spire.doc.documents;
using spire.doc.fields;
using system.collections.generic;
namespace spirexlsdemo
{
internal class program
{
static void main(string[] args)
{
// 创建一个新的document对象
document doc = new document();
// 从文件加载文档内容
doc.loadfromfile("示例2.docx");
// 获取文档的主体部分
body body = doc.sections[0].body;
// 获取主体部分的第一个段落
paragraph paragraph = body.paragraphs[0];
// 遍历段落中的子对象
for (int i = 0; i < paragraph.childobjects.count; i )
{
// 检查子对象是否为structuredocumenttaginline类型
if (paragraph.childobjects[i].documentobjecttype == documentobjecttype.structuredocumenttaginline)
{
// 将子对象转换为structuredocumenttaginline类型
structuredocumenttaginline structuredocumenttaginline = (structuredocumenttaginline)paragraph.childobjects[i];
// 检查文档标记的tag或alias属性是否为"text1"
if (structuredocumenttaginline.sdtproperties.tag == "text1" || structuredocumenttaginline.sdtproperties.alias == "text1")
{
// 遍历structuredocumenttaginline对象里的子对象
for (int j = 0; j < structuredocumenttaginline.childobjects.count; j )
{
// 检查子对象是否为textrange对象
if (structuredocumenttaginline.childobjects[j].documentobjecttype == documentobjecttype.textrange)
{
// 将子对象转换为textrange类型
textrange range = (textrange)structuredocumenttaginline.childobjects[j];
// 设置文本内容为指定内容
range.text = "word97-2003、word2007、word2010、word2013、word2016以及word2019";
}
}
}
// 检查文档标记的tag或alias属性是否为"logo1"
if (structuredocumenttaginline.sdtproperties.tag == "logo1" || structuredocumenttaginline.sdtproperties.alias == "logo1")
{
// 遍历structuredocumenttaginline对象里的子对象
for (int j = 0; j < structuredocumenttaginline.childobjects.count; j )
{
// 检查子对象是否为图片
if (structuredocumenttaginline.childobjects[j].documentobjecttype == documentobjecttype.picture)
{
// 将子对象转换为docpicture类型
docpicture docpicture = (docpicture)structuredocumenttaginline.childobjects[j];
// 加载指定图片
docpicture.loadimage("doc-net.png");
// 设置图片宽度和高度
docpicture.width = 100;
docpicture.height = 100;
}
}
}
}
}
// 将修改后的文档保存为新的文件
doc.savetofile("修改word文档的段落中的内容控件.docx", fileformat.docx2016);
// 释放document对象的资源
doc.dispose();
}
}
}
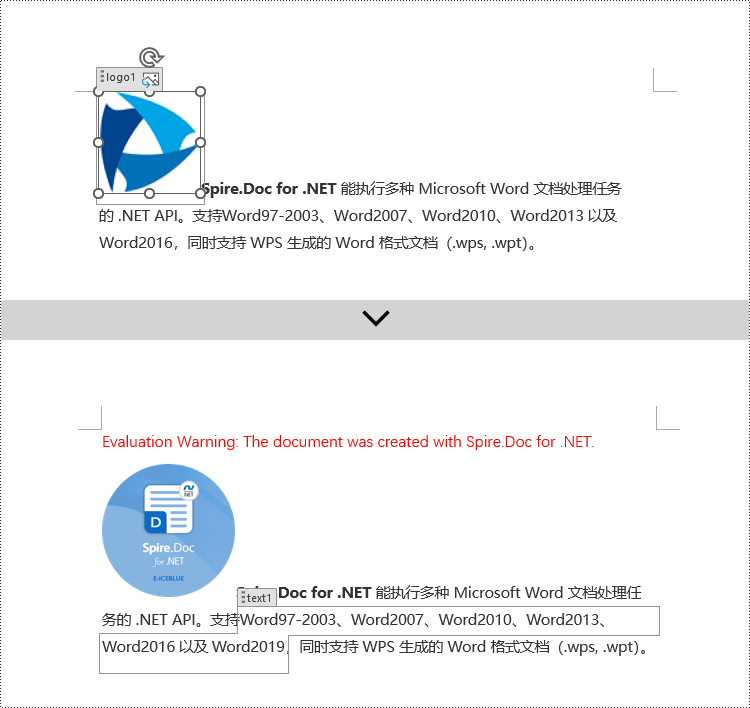
c# 修改表格行内容控件
在 spire.doc 中,表格行内容控件对象类型是 structuredocumenttagrow。需要遍历 table.childobjects 的子对象集合,查找到类型为 structuredocumenttagrow 的对象,然后对其进行修改。以下是详细的步骤:
- 创建一个 document 对象。
- 使用 document.loadfromfile() 方法加载一个文档。
- 使用 section.body 获取文档一个节的正文部分。
- 使用 body.tables[0] 获取正文部分的第一个表格。
- 遍历表格的子对象集合 table.childobjects,获取到类型为 structuredocumenttagrow 的子对象。
- 进入 structuredocumenttagrow.cells 表格行内容控件的单元格集合,然后对单元格内容执行相应的修改操作。
- 使用 document.savetofile() 方法保存到文档。
- c#
using spire.doc;
using spire.doc.documents;
using spire.doc.fields;
namespace spiredocdemo
{
internal class program
{
static void main(string[] args)
{
// 创建一个新的文档对象
document doc = new document();
// 从文件加载文档
doc.loadfromfile("示例3.docx");
// 获取文档正文部分
body body = doc.sections[0].body;
// 获取第一个表格
table table = (table)body.tables[0];
// 遍历表格中的子对象
for (int i = 0; i < table.childobjects.count; i )
{
// 判断子对象是否为structuredocumenttagrow类型
if (table.childobjects[i].documentobjecttype == documentobjecttype.structuredocumenttagrow)
{
// 将子对象转换为structuredocumenttagrow对象
structuredocumenttagrow structuredocumenttagrow = (structuredocumenttagrow)table.childobjects[i];
// 检查structuredocumenttagrow的tag或alias属性是否为"row1"
if (structuredocumenttagrow.sdtproperties.tag == "row1" || structuredocumenttagrow.sdtproperties.alias == "row1")
{
// 清空单元格中的段落
structuredocumenttagrow.cells[0].paragraphs.clear();
// 在单元格中添加一个段落,并设置文本
textrange textrange = structuredocumenttagrow.cells[0].addparagraph().appendtext("艺术");
textrange.characterformat.textcolor = system.drawing.color.blue;
}
}
}
// 将修改后的文档保存到文件
doc.savetofile("修改表格行内容控件.docx", fileformat.docx2016);
// 释放文档资源
doc.dispose();
}
}
}
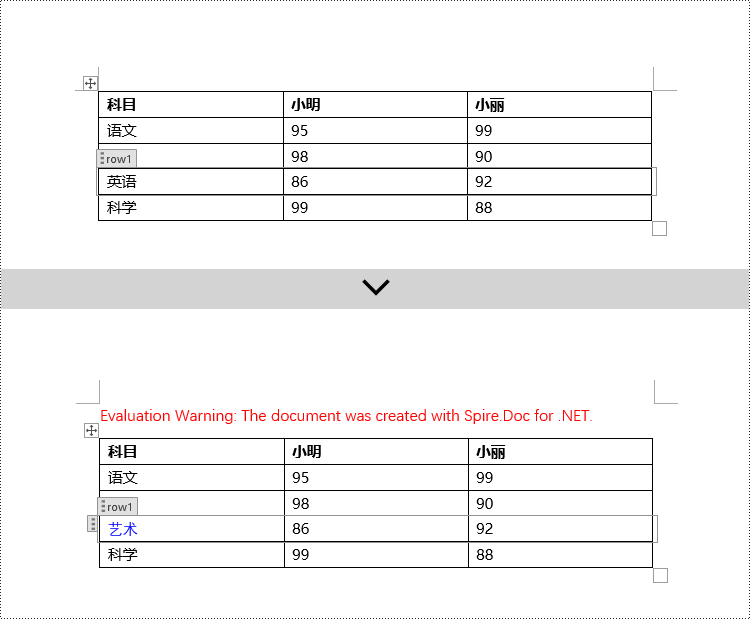
c# 修改表格单元格内容控件
在 spire.doc 中,表格单元格内容控件对象类型是 structuredocumenttagcell。需要遍历 tablerow.childobjects 的子对象集合,查找到类型为 structuredocumenttagcell 的对象,然后对其进行操作。以下是详细的步骤:
- 创建一个 document 对象。
- 使用 document.loadfromfile() 方法加载一个文档。
- 使用 section.body 获取文档一个节的正文部分。
- 使用 body.tables[0] 获取正文部分的第一个表格。
- 遍历表格行集合 table.rows,进入每一个 tablerow 对象。
- 遍历表格行的子对象集合 tablerow.childobjects,获取到类型为 structuredocumenttagcell 的子对象。
- 进入 structuredocumenttagcell.paragraphs 表格单元格内容控件的段落集合,然后对内容执行相应的修改操作。
- 使用 document.savetofile() 方法保存到文档。
- c#
using spire.doc;
using spire.doc.documents;
using spire.doc.fields;
namespace spiredocdemo
{
internal class program
{
static void main(string[] args)
{
// 创建一个新的文档对象
document doc = new document();
// 从文件加载文档
doc.loadfromfile("示例4.docx");
// 获取文档的正文部分
body body = doc.sections[0].body;
// 获取文档中的第一个表格
table table = (table)body.tables[0];
// 遍历表格的行
for (int i = 0; i < table.rows.count; i )
{
// 遍历每行中的子对象
for (int j = 0; j < table.rows[i].childobjects.count; j )
{
// 检查该子对象是否为structuredocumenttagcell
if (table.rows[i].childobjects[j].documentobjecttype == documentobjecttype.structuredocumenttagcell)
{
// 将子对象转换为structuredocumenttagcell类型
structuredocumenttagcell structuredocumenttagcell = (structuredocumenttagcell)table.rows[i].childobjects[j];
// 检查structuredocumenttagcell的tag或alias属性是否为"cell1"
if (structuredocumenttagcell.sdtproperties.tag == "cell1" || structuredocumenttagcell.sdtproperties.alias == "cell1")
{
// 清空单元格中的段落
structuredocumenttagcell.paragraphs.clear();
// 添加一个新段落,并在其中添加文本
textrange textrange = structuredocumenttagcell.addparagraph().appendtext("92");
textrange.characterformat.textcolor = system.drawing.color.blue;
}
}
}
}
// 将修改后的文档保存为新的文件
doc.savetofile("修改表格单元格内容控件.docx", fileformat.docx2016);
// 释放文档对象
doc.dispose();
}
}
}
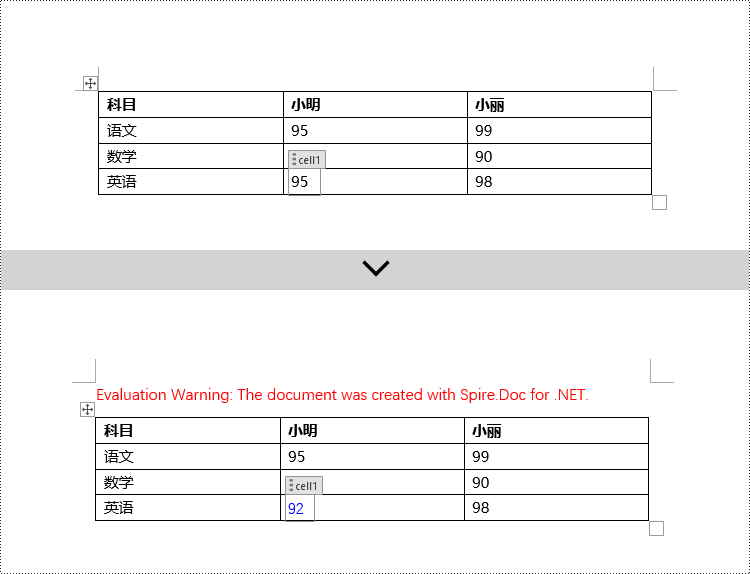
c# 修改表格单元格中的内容控件
这个案例展示的是修改在表格单元格的段落中的内容控件。需要先进入单元格中的段落集合 tablecell.pagragraphs,然后遍历每一个段落对象的子对象集合 paragraph.childobjects,查找到类型为 structuredocumenttaginline 的对象,然后对其进行修改。以下是详细的步骤:
- 创建一个 document 对象。
- 使用 document.loadfromfile() 方法加载一个文档。
- 使用 section.body 获取文档一个节的正文部分。
- 使用 body.tables[0] 获取正文部分的第一个表格。
- 遍历表格行集合 table.rows,进入每一个 tablerow 对象。
- 遍历单元格集合 tablerow.cells,进入每一个 tablecell 对象。
- 遍历单元格里的段落集合 tablecell.paragraphs,进入每一个 paragraph 对象。
- 遍历段落的子对象集合 paragraph.childobjects,查找到类型为 structuredocumenttaginline 的对象。
- 进入 structuredocumenttaginline.childobjects 子对象集合,根据子对象的类型执行相应的修改操作。
- 使用 document.savetofile() 方法保存到文档。
- c#
using spire.doc;
using spire.doc.documents;
using spire.doc.fields;
namespace spiredocdemo
{
internal class program
{
static void main(string[] args)
{
// 创建一个新的document对象
document doc = new document();
// 从文件加载文档内容
doc.loadfromfile("示例5.docx");
// 获取文档正文部分
body body = doc.sections[0].body;
// 获取第一个表格
table table = (table)body.tables[0];
// 遍历表格的行
for (int r = 0; r < table.rows.count; r )
{
// 遍历表格行中的单元格
for (int c = 0; c < table.rows[r].cells.count; c )
{
// 遍历单元格中的段落
for (int p = 0; p < table.rows[r].cells[c].paragraphs.count; p )
{
// 获取段落对象
paragraph paragraph = table.rows[r].cells[c].paragraphs[p];
// 遍历段落中的子对象
for (int i = 0; i < paragraph.childobjects.count; i )
{
// 判断子对象是否为structuredocumenttaginline类型
if (paragraph.childobjects[i].documentobjecttype == documentobjecttype.structuredocumenttaginline)
{
// 转换为structuredocumenttaginline对象
structuredocumenttaginline structuredocumenttaginline = (structuredocumenttaginline)paragraph.childobjects[i];
// 检查structuredocumenttaginline的tag或alias属性是否为"test1"
if (structuredocumenttaginline.sdtproperties.tag == "test1" || structuredocumenttaginline.sdtproperties.alias == "test1")
{
// 遍历structuredocumenttaginline的子对象
for (int j = 0; j < structuredocumenttaginline.childobjects.count; j )
{
// 判断子对象是否为textrange类型
if (structuredocumenttaginline.childobjects[j].documentobjecttype == documentobjecttype.textrange)
{
// 转换为textrange对象
textrange textrange = (textrange)structuredocumenttaginline.childobjects[j];
// 设置文本内容
textrange.text = "89";
//设置文本颜色
textrange.characterformat.textcolor = system.drawing.color.blue;
}
}
}
}
}
}
}
}
// 将修改后的文档保存为新文件
doc.savetofile("修改表格单元格中的内容控件.docx", fileformat.docx2016);
// 释放document对象资源
doc.dispose();
}
}
}
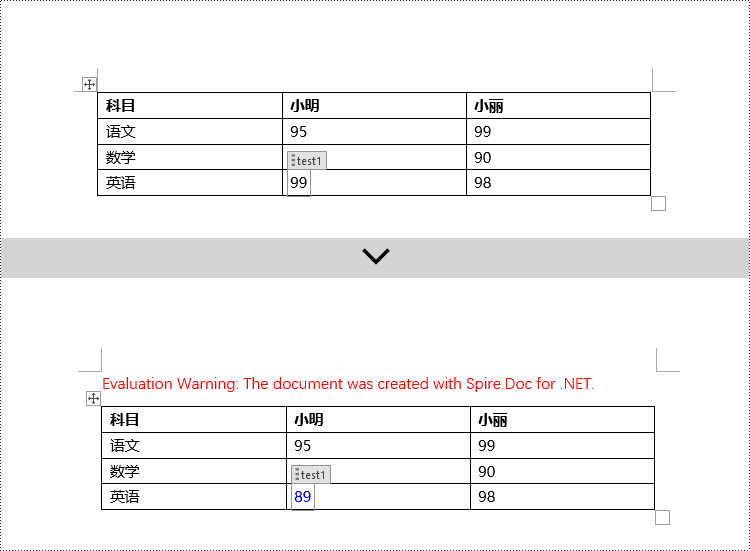
申请临时 license
如果您希望删除结果文档中的评估消息,或者摆脱功能限制,请该email地址已收到反垃圾邮件插件保护。要显示它您需要在浏览器中启用javascript。获取有效期 30 天的临时许可证。

